This article is in English, you can read a Mandarin(中文) translation here.
This series is split into 4 parts:
Part 1 — An introduction to AZTEC
Part 2 — Deploying AZTEC on Ganache
Part 3 — Constructing Proofs, Signing Flows and Key Management
Part 4 — Creating, Settling, & Streaming Confidential Assets
The demo dApp implements a confidential loan on Ethereum. The loan provides for the following functionality :
- A borrower can create a loan request with a confidential loan notional.
- A lender can request access to see the value of the loan notional.
- A lender can settle a loan request by transferring the notional to the borrower, the transfer notional should be confidential. The blockchain should verify that the notional amount and the settlement amount are equal.
- The borrower should be able to pay interest into an account that the lender can withdraw from. Any payments to the interest account should be confidential.
- The lender should be able to withdraw interest from the interest account as it accrues up to the last block time. The blockchain should verify the amount of interest the lender is withdrawing is correct, and the withdraw amount and the balance of the account should remain confidential.
- The lender should be able to mark a loan as defaulting if the interest account does not contain sufficient interest. The blockchain should validate that this is the case whilst keeping the total interest payed, the account balance and the loan’s notional confidential.
- The borrower should be able to repay the loan and any outstanding accrued interest at maturity. Both the interest and the notional repayment should remain confidential.
To build the above functionality, the dApp will combine two confidential assets, and the following proofs: Mint Proof, Join Split Proof, Bilateral Swap Proof, Dividend Proof, Private Range Proof.
Creating the Loan ZkAsset
As the loan is intended to be a fully private asset without a public equivalent, it will inherit from the reference EIP1724ZkAssetMintable.sol contract. In this case, the constructor is overridden with to create a fully private asset.
pragma solidity >= 0.5.0 <0.7.0;
import "@aztec/protocol/contracts/ERC1724/ZkAssetMintable.sol";
import "@aztec/protocol/contracts/libs/NoteUtils.sol";
import "@aztec/protocol/contracts/interfaces/IZkAsset.sol";
contract Loan is ZkAssetMintable {
using NoteUtils for bytes;
constructor(
address _aceAddress,
) public ZkAssetMintable(_aceAddress, address(0), 1, true, false) {
}
}
All AZTEC toolkits perform logical checks on note values. To perform a logical check, a note must first be created. In order for the loan’s notional to be confidential, it must be represented as a note in the loan’s note registry. As the initial supply of any note registry is zero, in a private asset the Mint Proof must be used to adjust the total supply and create new notes.
Step 1: Constructing the Mint Proof
Firstly, construct a proof using aztec.js.
const {
proofData,
} = aztec.proof.mint.encodeMintTransaction({
newTotalMinted: newTotalNote,
oldTotalMinted: oldTotalNote,
adjustedNotes: [loanNotionalNote],
senderAddress: loanDappContract.address,
});
Step 2
This proof can now be used to Mint the new notes inside the loan’s note registry. Only the owner of the note registry is permitted to call the confidentialMintmethod. In this case, a smart contract called the constructor of the loan ZkAsset. That contract is the owner of the ZkAsset note registry. This permits it to validate a supplied proof and process the resultant transfer instructions inside ACE.
Loan(loanId).confidentialMint(MINT_PROOF, bytes(_proofData));
The Settlement ZkAsset
The primary functions of the loan (primary settlement, interest payments and repayment) require value transfer. As this value transfer is required to be confidential, the settlement asset also needs to be a ZkAsset that implements EIP1724. The ZkAsset represents the currency the loan counter-parties will use to transact and is redeemable for a public ERC20 token e.g (DAI, CUSD).
Creating the settlement asset requires initialising the ZkAsset constructor with different parameters to the Loan ZkAsset. This tells ACE that this asset is linked to a public ERC20 token and the supply is not adjustable.
pragma solidity >= 0.5.0 <0.7.0;
import "@aztec/protocol/contracts/ERC1724/ZkAsset.sol";
contract ZKERC20 is ZkAsset {
constructor(
address _aceAddress,
address _erc20Address
) public ZkAsset(_aceAddress, address(_erc20Address), 1, false, true) {
}
}
Creating an AZTEC note in the note registry of the Settlement ZkAsset requires a transfer of sufficient ERC20 tokens into ACE equal to the notes value multiplied by a scaling factor. These tokens are owned by ACE in return for creating the desired note.
It is worth noting that creating notes of a ZkAsset with a linked public token has limited confidentiality. An observer of the blockchain can deduce the notes created in any given transaction, sum to the amount of ERC20 consumed. As such it is recommended to create multiple notes in one transaction, in order to help obfuscate the value of individual notes.
If full confidentiality is required for the settlement asset, a private ZkAsset with no public equivalent should be used. Here, AZTEC notes are issued on receipt of funds via bank transfer. The notes are still 1–1 backed with fiat, similar to a stable coin, but the note creation transaction preserves confidentiality as no public ERC20 tokens are consumed. Carbon Money are working on an implementation of this.
This demo assumes a fully private asset is not required and consuming ERC20 tokens is an acceptable solution.
Step 1:
The ACE contract is approved to spend ERC20 tokens on behalf of the token owner.
await settlementToken.approve(aceContract.address, value);
Step 2: Creating the proof
const {
proofData,
expectedOutput
} = aztec.proof.joinSplit.encodeJoinSplitTransaction({
inputNotes: [],
outputNotes: [Note1, Note2], // note values sum to kPublic
senderAddress: account.address,
inputNoteOwners: [],
publicOwner: account.address,
kPublic: -value,
validatorAddress: joinSplitContract.address,
});
A particular variant of the Join Split proof is required when interacting with public value. The proof has no inputNotes, the input is a public value of ERC20 represented by kPublic. This value is negative as it represents value being converted into an AZTEC note form, (if value was redeemed from note form, the value would be positive). The Join Split proof is validation that the sum of the output notes is equal to the value of kPublic.
The proof construction also requires the Ethereum addresses of the publicOwner (the owner of the tokens spent in this transaction) and the senderAddress (the account that will send this transaction to the ACE for validation), to be set.
Step 3: Approving ACE to spend Tokens
Any proof that results in the transfer of public value has to be first approved by the owner of the public tokens for it to be valid. This allows ACE to transfer the value of the tokens consumed in the proof and acts as an additional security measure when dealing with ERC20s.
await ACE.publicApprove(zkAsset.address, hashProof, kPublic, {
from: accounts[0],
});
Step 4: Relaying the transaction
When relaying proofs to ACE, the sender address specified in the proof must match the msg.sender of the account that calls ACE.validateProof().This prevents malicious actors snooping on the transaction pool from front running the execution of this proof.
(bytes memory _proofOutputs) = ACE.validateProof(JOIN_SPLIT_PROOF, address(this), _proofData);
Step 5: Processing Transfer Instructions
Successful proof validation will return an array of proof outputs. These proof outputs contain the state update instructions that allow a dApp to update a note registry.
_loanVariables.settlementToken.confidentialTransferFrom(JOIN_SPLIT_PROOF, _proof2Outputs.get(0));
Settling the loan
Once the loan ZkAsset and the settlement ZkAsset have been created, and each note registry populated with the initial notes, the loan is prepared for settlement. The diagram below shows the state of our dApp at this point and the swap that is required for settlement

To settle the loan the Bilateral Swap Proof is required. The borrower wishes to receive a note of the settlement ZkAsset equal to the loans notional multiplied by the loan price. The lender wishes to receive a note that represents 100% of the loan’s ownership register, in this case the notional note. Later on, this note will be used to claim interest and repayment at maturity. The ownership note can also be split and transferred should the lender wish to trade the loan.
Step 1 : Approving the settlement contract to spend notes
As the settlement transaction needs to be atomic, the transaction will be orchestrated by a smart contract. After a proof has been validated, ACE will only process the state updates (create or destroy notes) if the notes destroyed in a transaction have first been approved for spending by the note owner. The validation and processing of the Bilateral Swap proof must occur in an atomic transaction, otherwise, if one side of a transaction fails to approve the notes for spending, there is a chance one party will not receive their required ask in the swap. It is up to the dApp developer to ensure the correct permission logic is in place when calling functions within the AZTEC system. ACE will only validate the mathematical logic of a transaction, but does not know if a transaction should take place. In the case of loan settlement, the dApp should validate that the input notes have been approved by both the buyer and the seller and they are agree to the transfer.
In order for the transaction to process correctly, both the borrower and the lender need to approve the settlement contract to spend their respective notes.
const settlementSignature = signNote(
zkSettlementAsset.address,
settlementNoteHash,
loanId,
lender.privateKey);
await zkSettlementAsset.confidentialApprove(
settlementNoteHash,
loanId,
true,
settlementSignature,
{
from: lender.address,
});
Step 2: Constructing the proof
const {
proofData,
} = aztec.proof.bilateralSwap.encodeBilateralSwapTransaction({
inputNotes: [takerBid, takerAsk],
outputNotes: [makerAsk, makerBid],
senderAddress: loanId,
});
The proof requires 4 notes, and will validate the following logical statements:
- The takerBid note is equal to the makerAsk note.
- The takerAsk note is equal to the makerBid note.
Step 3: Relaying the Transaction and Updating State
When relaying proofs to ACE, the sender address specified in the proof must match the msg.sender of the account that calls ACE.validateProof().This prevents malicious actors snooping on the transaction pool from front running the execution of this proof.
Once validated, the proof outputs can be used to update the retrospective note registries. This will destroy the takerBid note and create the makerAsk note in the settlement ZkAsset note registry and destroy the makerBid note and create the takerAsk note in the loan ZkAsset note registry.
(bytes memory _proofOutputs) = ACE.validateProof(BILATERAL_SWAP_PROOF, address(this), _proofData);
(bytes memory _loanProofOutputs) = _proofOutputs.get(0);
(bytes memory _settlementProofOutputs) = _proofOutputs.get(1);
settlementZkAsset.confidentialTransferFrom(BILATERAL_SWAP_PROOF, _settlementProofOutputs);
loanZkAsset.confidentialTransferFrom(BILATERAL_SWAP_PROOF, _loanProofOutputs);
Thats it! The loan has been settled and all balances remain confidential.
Interest Streaming
AZTEC notes can be owned by smart contracts. This makes it is possible to construct complicated financial instruments using AZTEC. For the loan, we wish to create a system in which the lender can withdraw interest from an account as it accrues. Should the interest account contain insufficient collateral the lender should be able to mark the loan as defaulting and the smart contract transfer any security used as collateral to the lender.
To make interest streaming non-interactive from the borrowers point of view, the blockchain must validate the interest the lender is trying to withdraw is not greater than the currently accrued interest, and use this validation to ensure the correct amount of interest is then withdrawn. This flow is possible by combing the Dividend Proof and the Join Split proof. The Dividend Proof allows us to prove that one note is a ratio of another note plus a residual (to account for the quirks of solidity arithmetic).
Note1 * a = Note2 * b + Residual
If Note2 is set as the withdrawal note, the proof creator is incentivised to pick values of a and b such that the residual note is minimised. This enables Note2 to be expressed as a ratio of Note1 .
Note1 = Note2 * b/a
To apply this to the loan, a ratio must be found that expresses the AccruedInterest with respect to another note supplied by the smart contract in this case the notional.
This is possible with a little algebra:
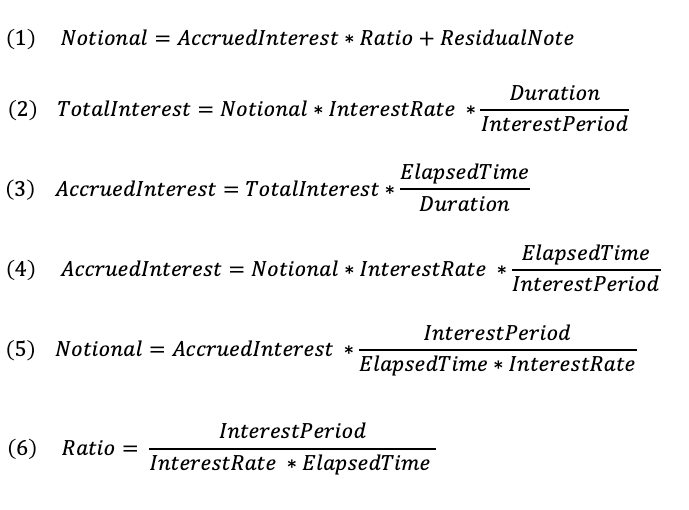
As a smart contract can set the values of ElapsedTime, InterestRate and InterestPeriod. The lender will only be able to construct a proof that will satisfy equation (1) if the value of AccruedInterest picked is correct up to the last block time.
If the Dividend Proof succeeds, the Accrued interest note that is used can be trusted and if supplied inside a subsequent valid Join Split proof, can be used to split the CurrentInterestBalance into the AccruedInterest plus a remainder note.
This process can be repeated for each block allowing the lender to withdraw interest as it accrues by the second. In each case, the blockchain will validate this correctness of the withdrawal.

Programatic Default — No Lawyers
Historically, should a borrower fail to pay interest on a loan or fail to pay back the loan at repayment, the lender would have to go through the courts to claim any collateral in lieu of repayment. Interest streaming allows the blockchain to validate a state of default and programatically transfer any collateral to the lender without the need for any arbitration, lawyers or courts.
To achieve this, two proofs are combined the Dividend Proof as used before to validate the currently accrued interest, and the Private Range Proof, to validate that the accrued interest is greater than the available balance inside the interest account.
Putting it all together — DEMO
The Loan dApp is available on github and can be cloned here.
Thanks for reading Part 4 of this series!